2021. 8. 20. 14:34ㆍDeep Learning
Binary Classification은 딥러닝에서 분류하고자하는 것이 0,1로 나타날 수있는 2가지일 경우 사용한다. 스팸판정에서 Spam[1], Ham[0], SNS에서 피드의 show[1], hide[0] 등에 사용된다.
1단계) Linear regression H(x) = Wx + b
2단계) Logistic/sigmoid function (sig(t))
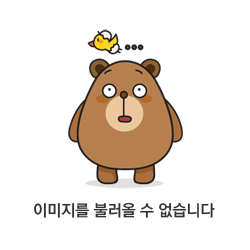
로지스틱 함수는 다음과 같다. 이 함수를 통해서 0,1로 mapping이 가능해진다. 로지스틱 함수를 씌우면 복잡해서 convex 가 보장되지 않는다는 문제점이 생긴다. 그래서 Linear Regression Model에서 사용했던 Gradient Descent Method는 사용될 수 없다.
Log 기반의 새로운 cost 함수를 사용해 분모의 지수승의 x를 꺼내 convex 형대로 만들어준다.
분류기 프로그래밍을 구현해보자
!pip install tensorflow==1.15
import tensorflow as tf
x_data = [ [1,2], [2,3], [3,1], [4,3], [5,3], [6,2]]
y_data = [ [0], [0],[0], [1], [1], [1] ] # 이진분류
X = tf.placeholder(tf.float32, shape=[None, 2])
Y = tf.placeholder(tf.float32, shape=[None, 1])
W = tf.Variable(tf.random_normal([2,1]))
b = tf.Variable(tf.random_normal([1]))
model = tf.sigmoid(tf.add(tf.matmul(X,W), b)) #모델을 0,1 사이로 나오게 합니다.
cost = tf.reduce_mean((-1)*Y*tf.log(model)+(-1)*(1-Y)*tf.log(1-model))
train = tf.train.GradientDescentOptimizer(0.01).minimize(cost)
prediction = tf.cast(model > 0.5 , dtype=tf.float32) #tf.cast 참이면 1, 거짓이면 0인 함수 #0.5보자 작으면 1, 크면 0이 도출됨.
accuaracy = tf.reduce_mean(tf.cast(tf.equal(prediction,Y),dtype=tf.float32)) #tf.equal 같은가? 함수 #정확도
with tf.Session() as sess:
sess.run(tf.global_variables_initializer())
# Tranining
for step in range(10001):
cost_val, train_val = sess.run([cost, train], feed_dict={X:x_data, Y:y_data})
print(step, cost_val)
# Testing
h, c, a = sess.run([model, prediction, accuaracy], feed_dict={X:x_data, Y:y_data})
print("\nModel: ", h, "\nCorrect: ", c, "\nAccuracy: ", a)
x_data, y_data 데이터를 통해 훈현하면서 W, b를 업데이트 하면서 0,1로 분류하는 모델을 만들고 정확도를 구했다.
결과는 다음과 같다.
Model: [[0.03229216] [0.16089834] [0.3122077 ] [0.7780485 ] [0.9374546 ] [0.9794598 ]]
Correct: [[0.] [0.] [0.] [1.] [1.] [1.]]
Accuracy: 1.0 (정확도는 100%)
reference
Install TensorFlow with pip
https://www.tensorflow.org/install/pip?hl=ko
Install TensorFlow with pip
TensorFlow 2 packages are available tensorflow —Latest stable release with CPU and GPU support (Ubuntu and Windows) tf-nightly —Preview build (unstable) . Ubuntu and Windows include GPU support . Older versions of TensorFlow For TensorFlow 1.x, CPU and
www.tensorflow.org
'Deep Learning' 카테고리의 다른 글
딥러닝_합성곱 신경망 (Convolutional Neural Network, CNN) (0) | 2021.08.26 |
---|---|
딥러닝_Softmax Classification (0) | 2021.08.21 |
딥러닝_Linear Regression Implementation (0) | 2021.08.19 |